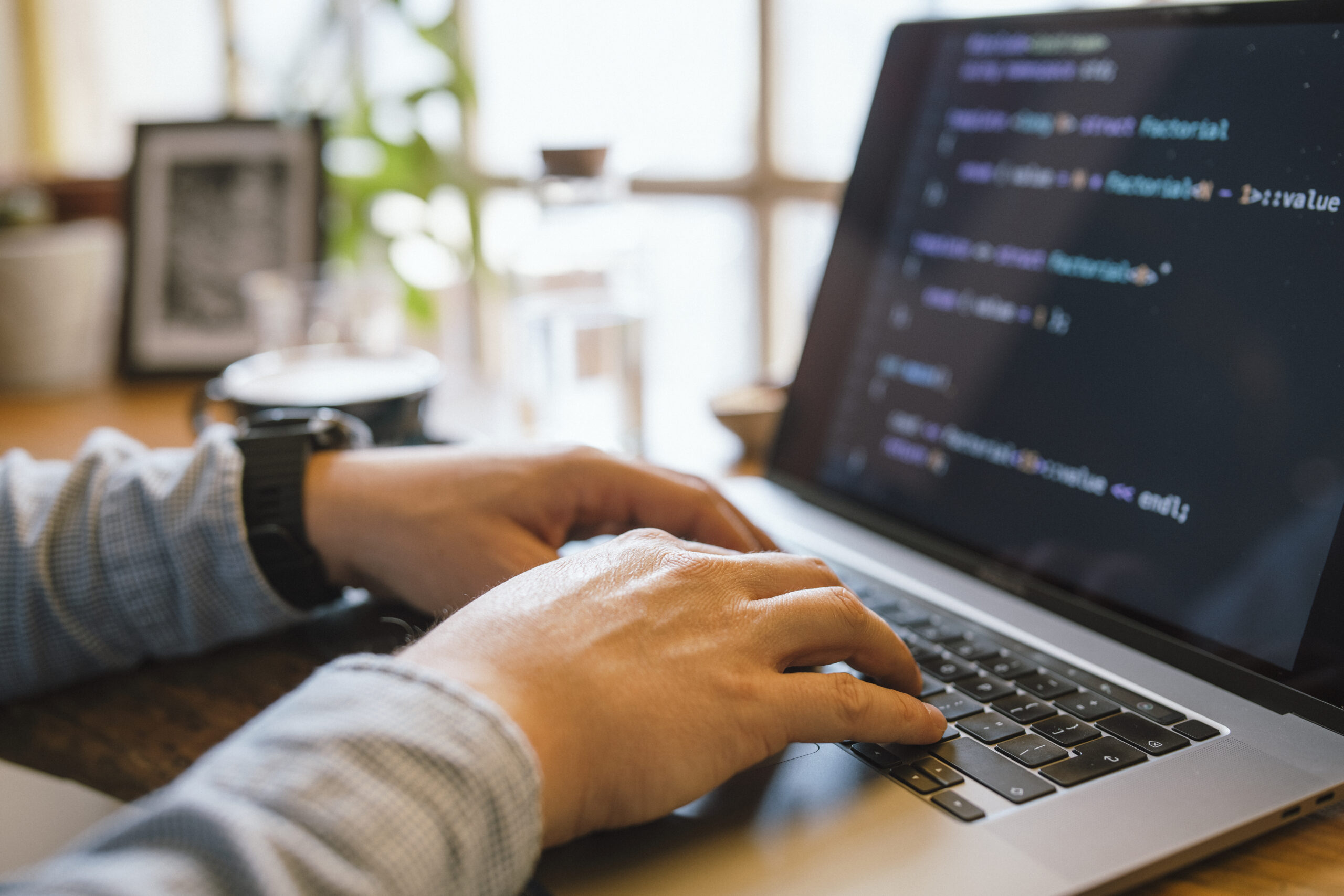
Debugging is Probably the most important — nevertheless generally overlooked — abilities within a developer’s toolkit. It's actually not almost correcting damaged code; it’s about being familiar with how and why things go Improper, and Finding out to Assume methodically to unravel complications competently. Whether you're a beginner or a seasoned developer, sharpening your debugging skills can conserve hours of aggravation and drastically boost your productiveness. Listed below are numerous methods to assist developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
Among the fastest means builders can elevate their debugging capabilities is by mastering the equipment they use daily. Even though composing code is a single A part of improvement, realizing how you can communicate with it effectively all through execution is Similarly essential. Fashionable progress environments appear Geared up with impressive debugging abilities — but several developers only scratch the floor of what these applications can perform.
Consider, for example, an Built-in Improvement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, step by code line by line, as well as modify code around the fly. When made use of effectively, they Allow you to notice precisely how your code behaves through execution, that's invaluable for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-finish developers. They permit you to inspect the DOM, watch network requests, look at serious-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can flip annoying UI challenges into manageable duties.
For backend or process-stage builders, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle about running processes and memory administration. Studying these equipment could possibly have a steeper Discovering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be relaxed with Variation Command methods like Git to comprehend code historical past, find the exact second bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications implies going further than default configurations and shortcuts — it’s about developing an intimate knowledge of your progress natural environment to make sure that when issues crop up, you’re not shed in the dark. The greater you understand your equipment, the more time you are able to invest solving the particular trouble as opposed to fumbling by means of the process.
Reproduce the issue
Probably the most critical — and often missed — steps in effective debugging is reproducing the trouble. Just before jumping into your code or building guesses, developers will need to make a steady environment or state of affairs wherever the bug reliably appears. Without reproducibility, fixing a bug results in being a video game of likelihood, frequently bringing about wasted time and fragile code changes.
The first step in reproducing a dilemma is collecting just as much context as is possible. Request questions like: What steps brought about the issue? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The more element you have, the a lot easier it turns into to isolate the precise problems under which the bug happens.
Once you’ve gathered ample information, try and recreate the issue in your neighborhood environment. This might mean inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider crafting automated exams that replicate the edge scenarios or state transitions concerned. These assessments not merely assistance expose the issue and also prevent regressions Later on.
From time to time, The difficulty might be ecosystem-particular — it would transpire only on certain working programs, browsers, or less than certain configurations. Working with applications like virtual machines, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these bugs.
Reproducing the condition isn’t only a phase — it’s a way of thinking. It necessitates tolerance, observation, in addition to a methodical approach. But when you can continuously recreate the bug, you're currently halfway to fixing it. Having a reproducible situation, You can utilize your debugging equipment extra effectively, test possible fixes safely, and communicate more clearly along with your crew or consumers. It turns an abstract complaint into a concrete obstacle — Which’s wherever builders prosper.
Examine and Recognize the Error Messages
Error messages in many cases are the most beneficial clues a developer has when a thing goes Incorrect. Rather than looking at them as disheartening interruptions, builders need to find out to treat mistake messages as immediate communications with the technique. They usually tell you exactly what transpired, the place it occurred, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the message thoroughly and in full. Numerous builders, particularly when under time force, glance at the 1st line and instantly begin making assumptions. But further in the mistake stack or logs might lie the legitimate root lead to. Don’t just copy and paste error messages into serps — study and have an understanding of them 1st.
Break the error down into components. Could it be a syntax error, a runtime exception, or a logic error? Will it level to a selected file and line quantity? What module or functionality triggered it? These issues can information your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Understanding to acknowledge these can drastically accelerate your debugging system.
Some errors are imprecise or generic, and in Individuals scenarios, it’s crucial to examine the context through which the mistake happened. Verify similar log entries, input values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings either. These typically precede much larger concerns and supply hints about probable bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, encouraging you pinpoint issues quicker, minimize debugging time, and become a far more successful and self-assured developer.
Use Logging Sensibly
Logging is One of the more powerful applications in a very developer’s debugging toolkit. When made use of effectively, it offers serious-time insights into how an software behaves, encouraging you understand what’s happening beneath the hood with no need to pause execution or phase throughout the code line by line.
A superb logging approach begins with being aware of what to log and at what degree. Frequent logging degrees include things like DEBUG, Details, WARN, ERROR, and Lethal. Use DEBUG for in-depth diagnostic information and facts through progress, Facts for typical gatherings (like prosperous start off-ups), WARN for potential challenges that don’t crack the appliance, Mistake for true issues, and FATAL in the event the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount of logging can obscure vital messages and decelerate your program. Focus on vital functions, state variations, enter/output values, and significant selection details with your code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to keep track of how variables evolve, what problems are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about harmony and clarity. Which has a effectively-considered-out logging approach, it is possible to reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the All round maintainability and trustworthiness within your code.
Consider Similar to a Detective
Debugging is not just a technical activity—it is a method of investigation. To effectively recognize and correct bugs, builders should technique the method similar to a detective resolving a secret. This state of mind aids break down intricate concerns into workable parts and adhere to clues logically to uncover the root result in.
Start off by collecting proof. Consider the indicators of the situation: error messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, acquire as much pertinent data as it is possible to with no leaping to conclusions. Use logs, exam conditions, and person stories to piece jointly a transparent image of what’s taking place.
Subsequent, type hypotheses. Request oneself: What could possibly be creating this behavior? Have any changes recently been built to your codebase? Has this situation transpired ahead of beneath comparable circumstances? The intention will be to slim down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Make an effort to recreate the challenge inside a managed setting. Should you suspect a specific functionality or element, isolate it and verify if The problem persists. Like a detective conducting interviews, talk to your code thoughts and Permit the outcomes guide you closer to the reality.
Pay out shut consideration to little facts. Bugs usually conceal in the minimum expected spots—like a lacking semicolon, an off-by-one mistake, or a race affliction. Be thorough and patient, resisting the urge to patch The problem with out thoroughly comprehending it. Momentary fixes might cover the actual difficulty, just for it to resurface later.
Last of all, preserve notes on Anything you attempted and figured out. Equally as detectives log their investigations, documenting your debugging method can help save time for long term troubles and assistance Other individuals understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed challenges in complex techniques.
Write Tests
Composing assessments is among the simplest ways to enhance your debugging capabilities and Over-all enhancement performance. Exams not merely enable capture bugs early but will also function a security Web that offers you confidence when creating adjustments in your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint accurately where and when a problem occurs.
Get started with device assessments, which focus on individual functions or modules. These small, isolated checks can immediately expose irrespective of whether a selected bit of logic is Doing work as anticipated. Whenever a examination fails, you quickly know in which to search, considerably decreasing the time used debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear just after previously remaining fastened.
Following, integrate integration checks and conclusion-to-conclude tests into your workflow. These assistance be sure that a variety of elements of your application work alongside one another efficiently. They’re especially useful for catching bugs that come about in sophisticated systems with many elements or services interacting. If a thing breaks, your exams can tell you which Element of the pipeline failed and less than what problems.
Writing assessments also forces you to Assume critically about your code. To check a function thoroughly, you will need to know its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to raised code construction and less bugs.
When debugging a difficulty, composing a failing exam that reproduces the bug may be a strong first step. When the exam fails constantly, you could concentrate on repairing the bug and check out your check move when The difficulty is resolved. This strategy makes certain that the identical bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the disheartening guessing sport into a structured and predictable course of action—encouraging you catch a lot more bugs, more rapidly plus more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to become immersed in the issue—watching your display check here screen for hrs, hoping Alternative after Answer. But The most underrated debugging instruments is actually stepping absent. Having breaks helps you reset your mind, decrease aggravation, and often see the issue from a new perspective.
When you're as well close to the code for too long, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code that you just wrote just hrs earlier. Within this state, your Mind results in being fewer successful at challenge-fixing. A brief stroll, a coffee break, or simply switching to a unique undertaking for 10–15 minutes can refresh your focus. Lots of builders report obtaining the root of a problem when they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially in the course of lengthier debugging classes. Sitting in front of a display screen, mentally stuck, is don't just unproductive and also draining. Stepping absent permits you to return with renewed energy in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a good guideline would be to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment crack. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily below limited deadlines, however it actually contributes to faster and simpler debugging Ultimately.
In brief, getting breaks isn't a sign of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and aids you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of resolving it.
Discover From Just about every Bug
Every bug you experience is much more than simply A short lived setback—It is a chance to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each can educate you a thing important if you take some time to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of critical thoughts once the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Create more robust coding practices relocating forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or preserve a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or prevalent issues—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug using your peers can be In particular strong. No matter whether it’s through a Slack information, a short write-up, or A fast information-sharing session, assisting Many others steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, some of the finest developers are certainly not the ones who generate excellent code, but those who continually learn from their blunders.
Eventually, Each and every bug you take care of adds a whole new layer to your ability established. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, more capable developer thanks to it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and patience — nevertheless the payoff is big. It will make you a more productive, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at That which you do.